Single Transfers
In a nutshell
Send money to your customers by using the Transfer API
You can transfer money in four easy steps:
- Create a transfer recipient
- Generate a transfer reference
- Initiate a transfer
- Listen for transfer status
Before you begin!
To send money on Paystack, you need API keys to authenticate your transfers. You can find your keys on the Paystack Dashboard under Settings → API Keys & Webhooks.
Create a transfer recipient
A transfer recipient is a beneficiary on your integration that you can send money to. Before sending money to your customer, you need to collect their details first, then use their details to create a transfer recipient. We support different recipients in different countries:
Type | Description | Currency |
---|---|---|
nuban | This means the Nigerian Uniform Bank Account Number. It represents bank accounts in Nigeria. | NGN |
mobile_money | Mobile Money or MoMo is an account tied to a mobile number. It is currently supported in Ghana and Kenya. | GHS/KES |
basa | This means the Banking Association South Africa. It represents bank accounts in South Africa | ZAR |
authorization | This is a unique code that represents a customer’s card. We return an authorization code after a user makes a payment with their card. | All |
The recipient_code
from the data object is the unique identifier for a user and would be used to make transfers to that customer This code
should be saved with the customer's records in your database.
Generate a transfer reference
A transfer reference is a unique identifier that lets you track, manage and reconcile each transfer request made on your integration. Transfer references allow you to prevent double crediting as you can retry a non-conclusive transfer rather than initiate a new request.
In order to take advantage of a transfer reference, you need to generate and provide it for every request. When you don’t provide a transfer reference, Paystack generates one for you but this defeats the purpose of the transfer reference.
We recommend generating a v4 UUID reference of no more than 100 characters. However, if you prefer implementing your own logic, you should ensure your reference contains at least 16 alphanumeric characters.
1{2 "source": "balance",3 "reason": "Savings",4 "amount": 30000,5 "reference": "your-unique-reference",6 "recipient": "RCP_1a25w1h3n0xctjg"7}
Initiate a transfer
To send money to a customer, you make a POST
request to the Initate TransferAPI, passing the reference
and recipient_code
previously created.
Disabling OTP
When building a fully automated system, you might need to disable OTP for transfers. You can disable OTP from the Preferences tab on the Paystack Dashoard. You should uncheck the Confirm transfers before sending checkbox as shown in the image below.
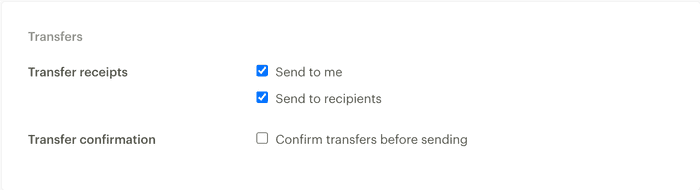
1curl https://api.paystack.co/transfer2-H "Authorization: Bearer YOUR_SECRET_KEY"3-H "Content-Type: application/json"4-d '{ "source": "balance",5 "amount": "37800",6 "reference": "your-unique-reference",7 "recipient": "RCP_t0ya41mp35flk40",8 "reason": "Holiday Flexing"9 }'10-X POST11
1{2 "status": true,3 "message": "Transfer has been queued",4 "data": {5 "reference": "your-unique-reference",6 "integration": 428626,7 "domain": "test",8 "amount": 37800,9 "currency": "NGN",10 "source": "balance",11 "reason": "Holiday Flexing",12 "recipient": 6788170,13 "status": "success",14 "transfer_code": "TRF_fiyxvgkh71e717b",15 "id": 23070321,16 "createdAt": "2020-05-13T14:22:49.687Z",17 "updatedAt": "2020-05-13T14:22:49.687Z"18 }19}
When you send this request, if there are no errors, the response comes back with a pending status, while the transfer is being processed.
Retrying a transfer
If there is an error with the transfer request, kindly retry the transaction with the same reference
in order to avoid double crediting. If a new reference
is used, the transfer would be treated as a new request.
Test transfers always return success, because there is no processing involved. The live transfers processing usually take between a few seconds and a few minutes. When it's done processing, a notification is sent to your webhook URL.
Verify a transfer
When a transfer is initiated, it could take a few seconds or minutes to be processed. This is why we recommend relying on webhooks for verification as opposed to polling.
Receiving Notifications
In order to receive notifications, you need to implement a webhook URL and set the webhook URL on your Paystack Dashboard.
Once a transfer is processed, we send the final status of the transfer as a POST
request to your webhook URL.
Event | Description |
---|---|
transfer.success | This is sent when the transfer is successful |
transfer.failed | This is sent when the transfer fails |
transfer.reversed | This is sent when we refund a previously debited amount for a transfer that couldn’t be completed |
- Transfer Successful
- Transfer Failed
- Transfer Reversed
1{2 "event": "transfer.success",3 "data": {4 "amount": 30000,5 "currency": "NGN",6 "domain": "test",7 "failures": null,8 "id": 37272792,9 "integration": {10 "id": 463433,11 "is_live": true,12 "business_name": "Boom Boom Industries NG"13 },14 "reason": "Have fun...",15 "reference": "1jhbs3ozmen0k7y5efmw",16 "source": "balance",17 "source_details": null,18 "status": "success",19 "titan_code": null,20 "transfer_code": "TRF_wpl1dem4967avzm",21 "transferred_at": null,22 "recipient": {23 "active": true,24 "currency": "NGN",25 "description": "",26 "domain": "test",27 "email": null,28 "id": 8690817,29 "integration": 463433,30 "metadata": null,31 "name": "Jack Sparrow",32 "recipient_code": "RCP_a8wkxiychzdzfgs",33 "type": "nuban",34 "is_deleted": false,35 "details": {36 "account_number": "0000000000",37 "account_name": null,38 "bank_code": "011",39 "bank_name": "First Bank of Nigeria"40 },41 "created_at": "2020-09-03T12:11:25.000Z",42 "updated_at": "2020-09-03T12:11:25.000Z"43 },44 "session": {45 "provider": null,46 "id": null47 },48 "created_at": "2020-10-26T12:28:57.000Z",49 "updated_at": "2020-10-26T12:28:57.000Z"50 }51}
The response for a transfer also contains a unique transfer code to identify this transfer. You can use this code to call the Fetch TransferAPI endpoint to get the status and details of the transfer.